Debugging Tests
The Playwright inspector is a great tool to help with debugging. It opens up a browser window highlighting the selectors as you step through each line of the test. You can also use the explore button to find other available selectors which you can then copy into your test file and rerun your tests to see if it passes. For debugging selectors, see here.
Playwright Inspector
Playwright Inspector is a GUI tool that helps authoring and debugging Playwright scripts. That's our default recommended tool for scripts troubleshooting.
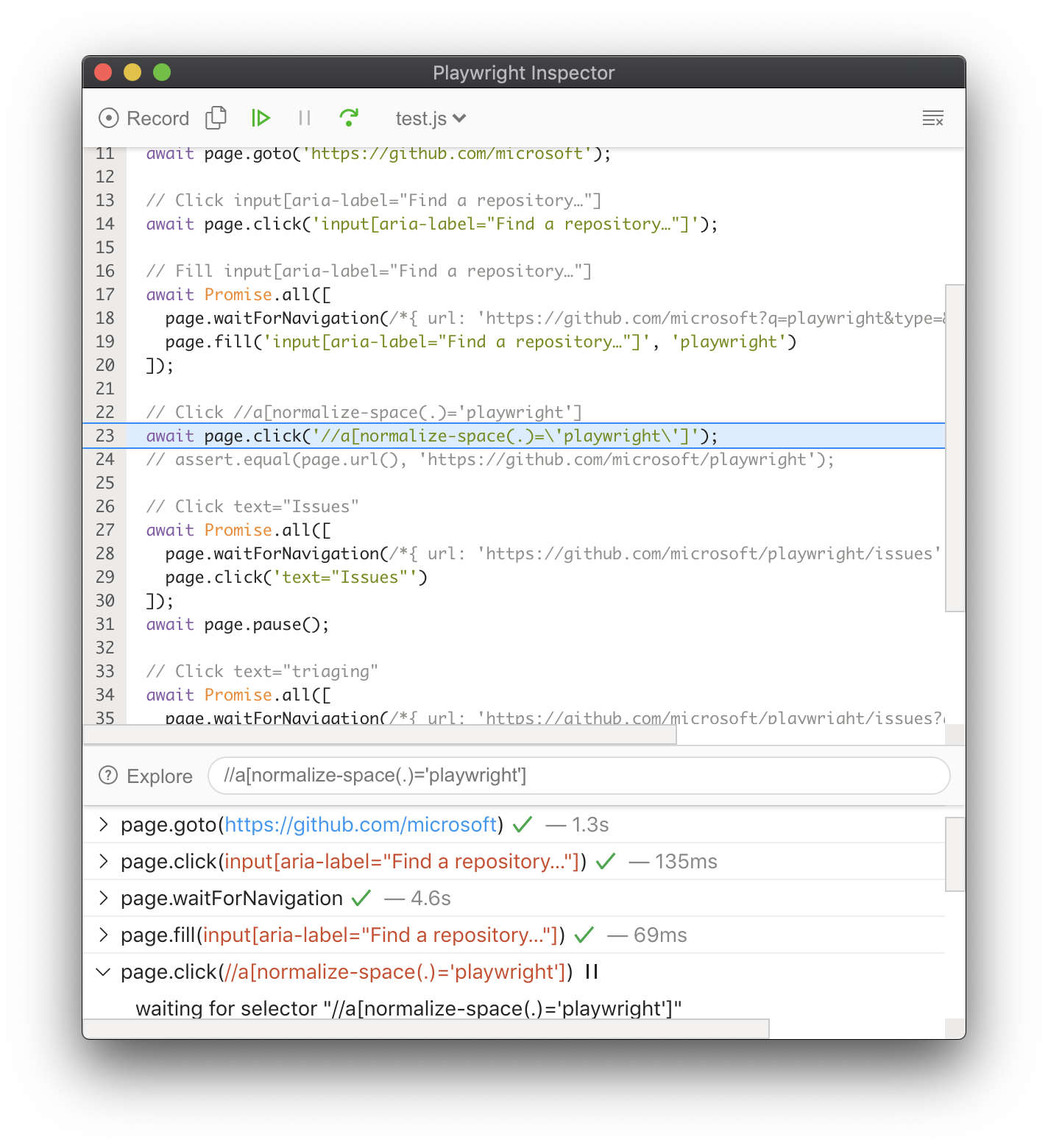
There are several ways of opening Playwright Inspector:
--debug
Debugging all Tests
npx playwright test --debug
Debugging one test
npx playwright test example --debug
PWDEBUG
Set the PWDEBUG
environment variable to run your scripts in debug mode. This configures Playwright for debugging and opens the inspector.
- Bash
- PowerShell
- Batch
PWDEBUG=1 npx playwright test
$env:PWDEBUG=1
npx playwright test
set PWDEBUG=1
npx playwright test
Additional useful defaults are configured when PWDEBUG=1
is set:
- Browsers launch in the headed mode
- Default timeout is set to 0 (= no timeout)
Using PWDEBUG=console
will configure the browser for debugging in Developer tools console:
- Runs headed: Browsers always launch in headed mode
- Disables timeout: Sets default timeout to 0 (= no timeout)
- Console helper: Configures a
playwright
object in the browser to generate and highlight Playwright selectors. This can be used to verify text or composite selectors.
- Bash
- PowerShell
- Batch
PWDEBUG=console npx playwright test
$env:PWDEBUG="console"
npx playwright test
set PWDEBUG=console
npx playwright test
page.pause
Call page.pause() method from your script when running in headed browser.
// Pause on the following line.
await page.pause();
Use open
or codegen
commands in the Playwright CLI:
npx playwright codegen wikipedia.org
Stepping through the Playwright script
The Inspector opens up a browser window highlighting the selectors as you step through each line of the test. Use the explore button to find other available selectors which you can then copy into your test file and rerun your tests to see if they pass.

Use the toolbar to play the test or step over each action using the "Step over" action (keyboard shortcut: F10
) or resume script without further pauses (F8
):
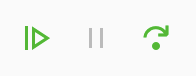
Now we know what action is about to be performed and we can look into the details on that action. For example, when stopped on an input action such as click
, the exact point Playwright is about to click is highlighted with the large red dot on the inspected page:
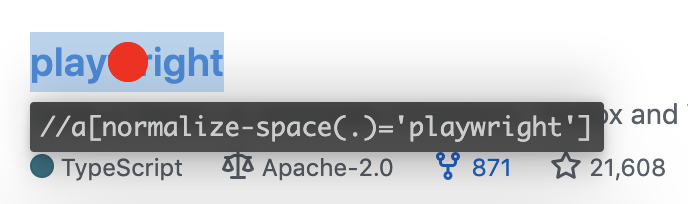
Actionability Logs
By the time Playwright has paused on that click action, it has already performed actionability checks that can be found in the log:
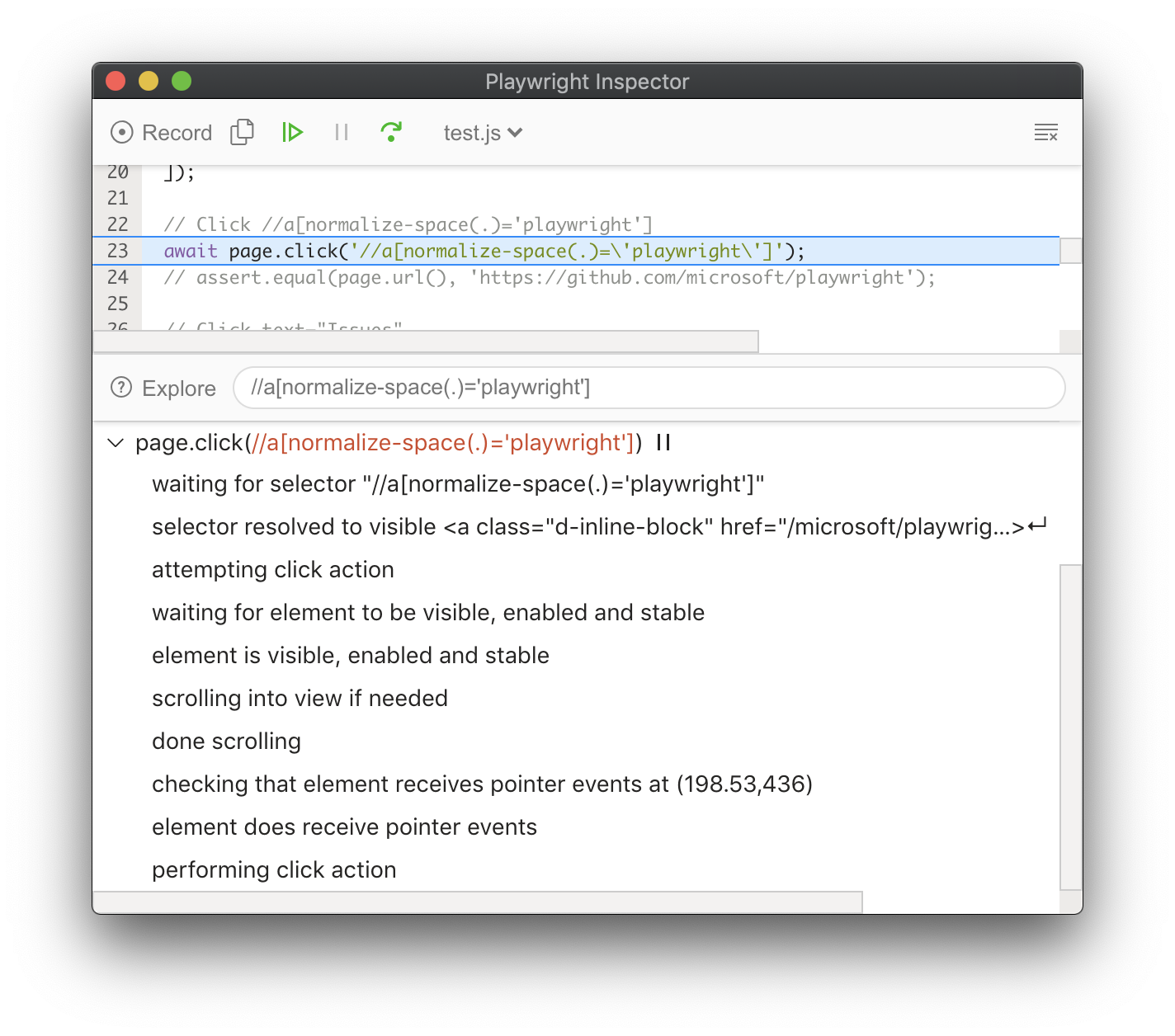
If actionability can't be reached, it'll show action as pending:
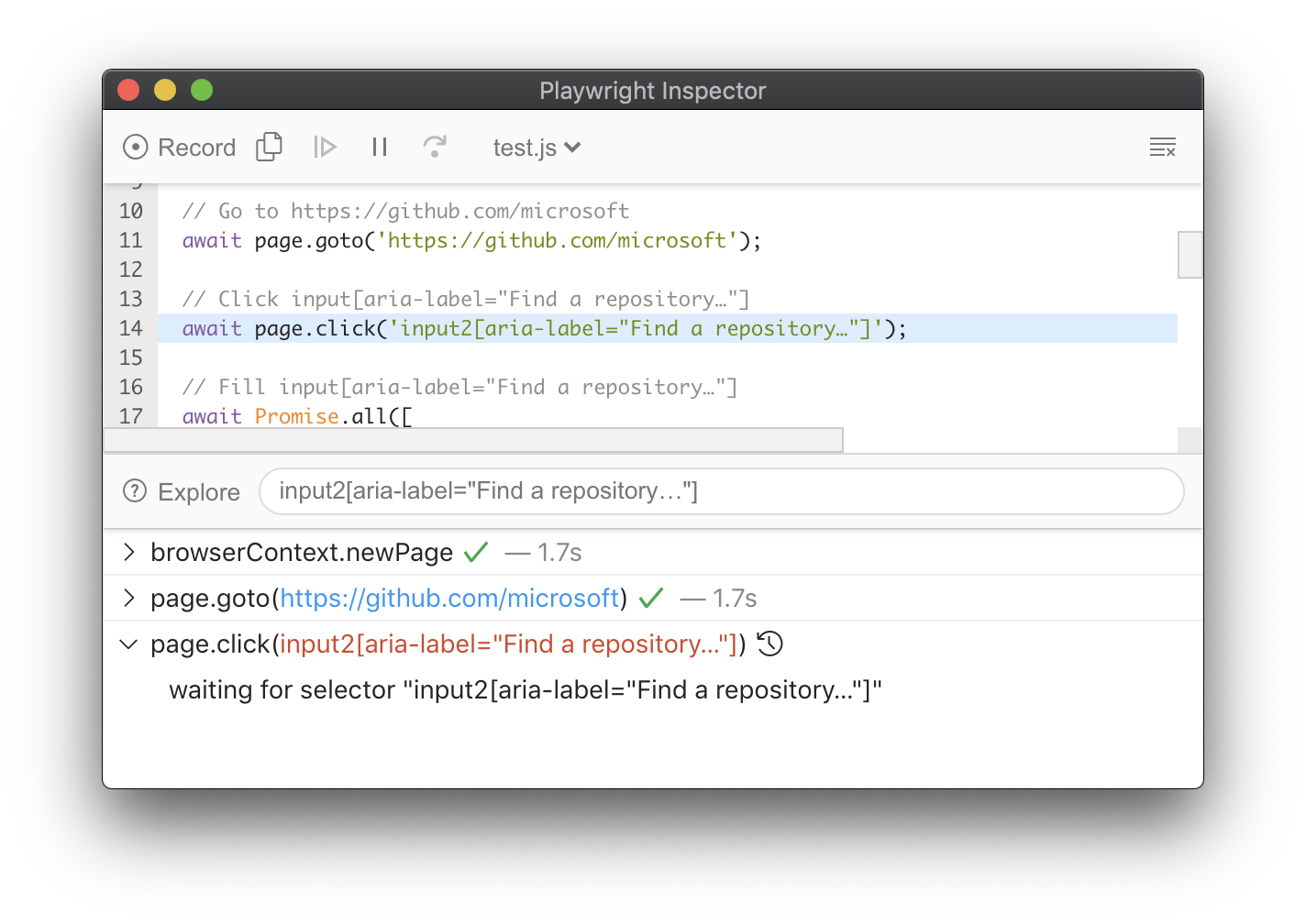
Exploring selectors
Use the Explore button to hover over an element on the page and explore it's selector by clicking on it. You can then copy this selector into your tests and rerun your tests to see if they now pass with this selector. You can also debug selectors, checkout our debugging selectors guide for more details.
Browser Developer Tools
You can use browser developer tools in Chromium, Firefox and WebKit while running a Playwright script in headed mode. Developer tools help to:
- Inspect the DOM tree and find element selectors
- See console logs during execution (or learn how to read logs via API)
- Check network activity and other developer tools features
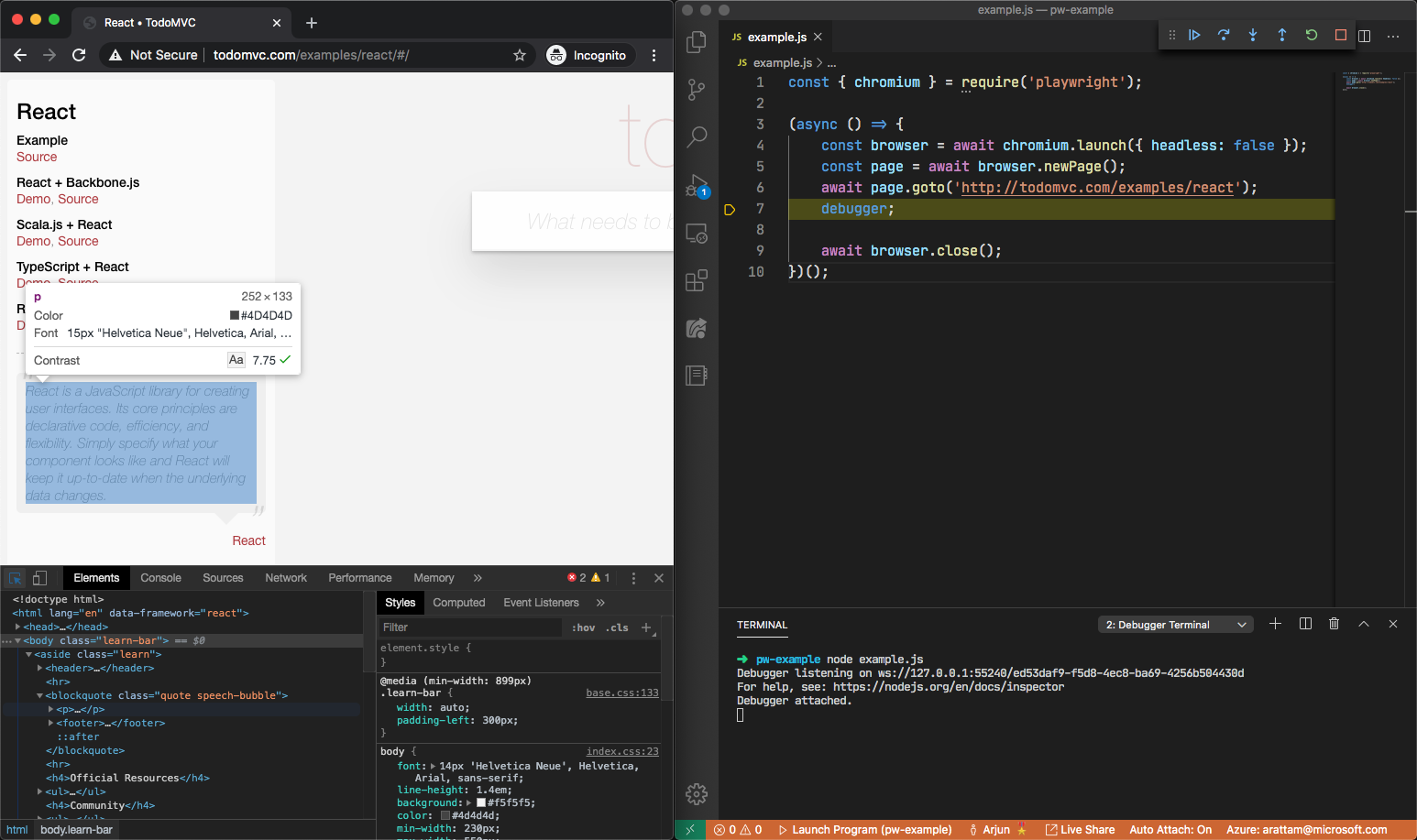
Using a page.pause() method is an easy way to pause the Playwright script execution and inspect the page in Developer tools. It will also open Playwright Inspector to help with debugging.
For Chromium: you can also open developer tools through a launch option.
await chromium.launch({ devtools: true });
For WebKit: launching WebKit Inspector during the execution will prevent the Playwright script from executing any further.
Headed mode
Playwright runs browsers in headless mode by default. To change this behavior, use headless: false
as a launch option. You can also use the slowMo
option to slow down execution and follow along while debugging.
await chromium.launch({ headless: false, slowMo: 100 }); // or firefox, webkit