Command line tools
Playwright comes with the command line tools.
Usage
playwright
Install browsers
Playwright can install supported browsers.
# Running without arguments will install default browsers
playwright install
You can also install specific browsers by providing an argument:
# Install WebKit
playwright install webkit
See all supported browsers:
playwright install --help
Install system dependencies
System dependencies can get installed automatically. This is useful for CI environments.
# See command help
playwright install-deps
You can also install the dependencies for a single browser only by passing it as an argument:
playwright install-deps chromium
It's also possible to combine install-deps
with install
and install by that the browsers and OS dependencies with a single command. This would do both for Chromium, but you can also leave it out.
playwright install --with-deps chromium
Generate code
playwright codegen wikipedia.org
Run codegen
and perform actions in the browser. Playwright CLI will generate JavaScript code for the user interactions. codegen
will attempt to generate resilient text-based selectors.
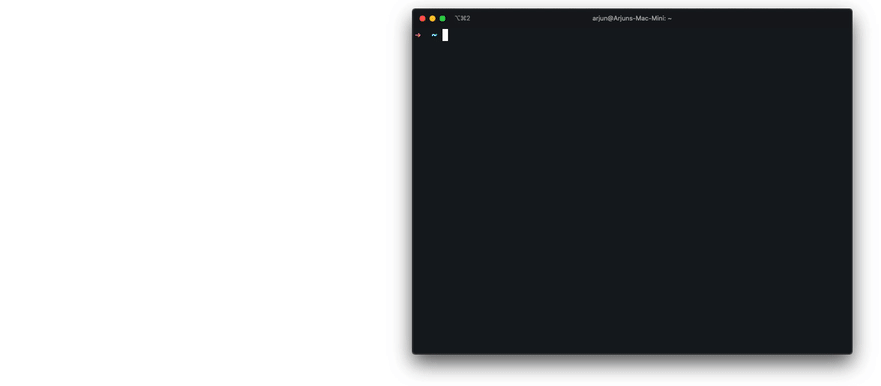
Preserve authenticated state
Run codegen
with --save-storage
to save cookies and localStorage at the end. This is useful to separately record authentication step and reuse it later.
playwright codegen --save-storage=auth.json
# Perform authentication and exit.
# auth.json will contain the storage state.
Run with --load-storage
to consume previously loaded storage. This way, all cookies and localStorage will be restored, bringing most web apps to the authenticated state.
playwright open --load-storage=auth.json my.web.app
playwright codegen --load-storage=auth.json my.web.app
# Perform actions in authenticated state.
Codegen with custom setup
If you would like to use codegen in some non-standard setup (for example, use browser_context.route(url, handler, **kwargs)), it is possible to call page.pause() that will open a separate window with codegen controls.
- Sync
- Async
from playwright.sync_api import sync_playwright
with sync_playwright() as p:
# Make sure to run headed.
browser = p.chromium.launch(headless=False)
# Setup context however you like.
context = browser.new_context() # Pass any options
context.route('**/*', lambda route: route.continue_())
# Pause the page, and start recording manually.
page = context.new_page()
page.pause()
import asyncio
from playwright.async_api import async_playwright
async def main():
async with async_playwright() as p:
# Make sure to run headed.
browser = await p.chromium.launch(headless=False)
# Setup context however you like.
context = await browser.new_context() # Pass any options
await context.route('**/*', lambda route: route.continue_())
# Pause the page, and start recording manually.
page = await context.new_page()
await page.pause()
asyncio.run(main())
Open pages
With open
, you can use Playwright bundled browsers to browse web pages. Playwright provides cross-platform WebKit builds that can be used to reproduce Safari rendering across Windows, Linux and macOS.
# Open page in Chromium
playwright open example.com
# Open page in WebKit
playwright wk example.com
Emulate devices
open
can emulate mobile and tablet devices from the playwright.devices
list.
# Emulate iPhone 11.
playwright open --device="iPhone 11" wikipedia.org
Emulate color scheme and viewport size
# Emulate screen size and color scheme.
playwright open --viewport-size=800,600 --color-scheme=dark twitter.com
Emulate geolocation, language and timezone
# Emulate timezone, language & location
# Once page opens, click the "my location" button to see geolocation in action
playwright open --timezone="Europe/Rome" --geolocation="41.890221,12.492348" --lang="it-IT" maps.google.com
Inspect selectors
During open
or codegen
, you can use following API inside the developer tools console of any browser.
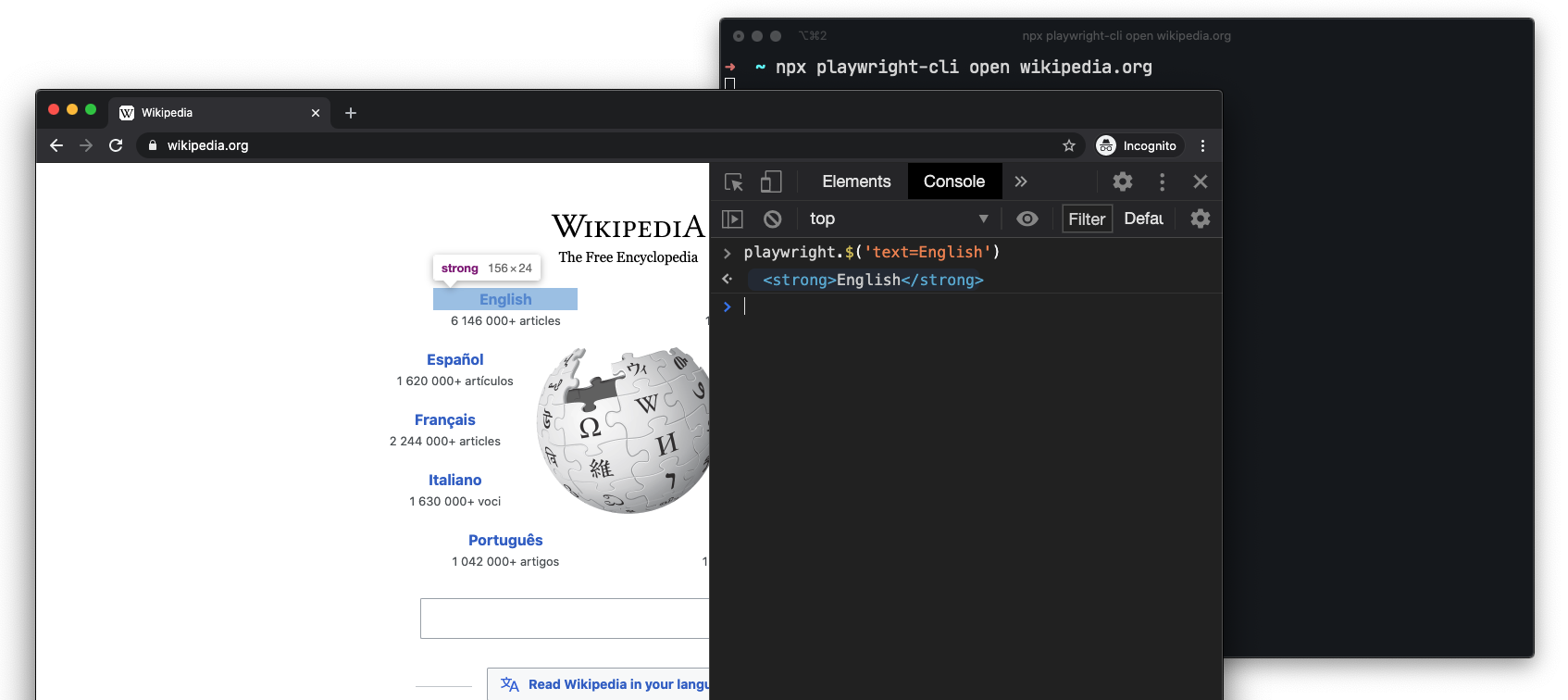
playwright.$(selector)
Query Playwright selector, using the actual Playwright query engine, for example:
playwright.$$(selector)
Same as playwright.$
, but returns all matching elements.
playwright.inspect(selector)
Reveal element in the Elements panel (if DevTools of the respective browser supports it).
playwright.locator(selector)
Query Playwright element using the actual Playwright query engine, for example:
playwright.selector(element)
Generates selector for the given element.
Take screenshot
# See command help
playwright screenshot --help
# Wait 3 seconds before capturing a screenshot after page loads ('load' event fires)
playwright screenshot \
--device="iPhone 11" \
--color-scheme=dark \
--wait-for-timeout=3000 \
twitter.com twitter-iphone.png
# Capture a full page screenshot
playwright screenshot --full-page en.wikipedia.org wiki-full.png
Generate PDF
PDF generation only works in Headless Chromium.
# See command help
playwright pdf https://en.wikipedia.org/wiki/PDF wiki.pdf