Debugging Selectors
Playwright will throw a timeout exception like locator.click: Timeout 30000ms exceeded
when an element does not exist on the page. There are multiple ways of debugging selectors:
- Playwright Inspector to step over each Playwright API call to inspect the page.
- Browser DevTools to inspect selectors with the DevTools element panel.
- Trace Viewer to see what the page looked like during the test run.
- Verbose API logs shows actionability checks when locating the element.
Using Playwright Inspector
Open the Playwright Inspector and click the Explore
button to hover over elements in the screen and click them to automatically generate selectors for those elements. To verify where selector points, paste it into the inspector input field:

Using DevTools
You can also use the following API inside the Developer Tools Console of any browser.
When running in Debug Mode with PWDEBUG=console
, a playwright
object is available in Developer tools console.
- Run with
PWDEBUG=console
- Setup a breakpoint to pause the execution
- Open the console panel in browser developer tools
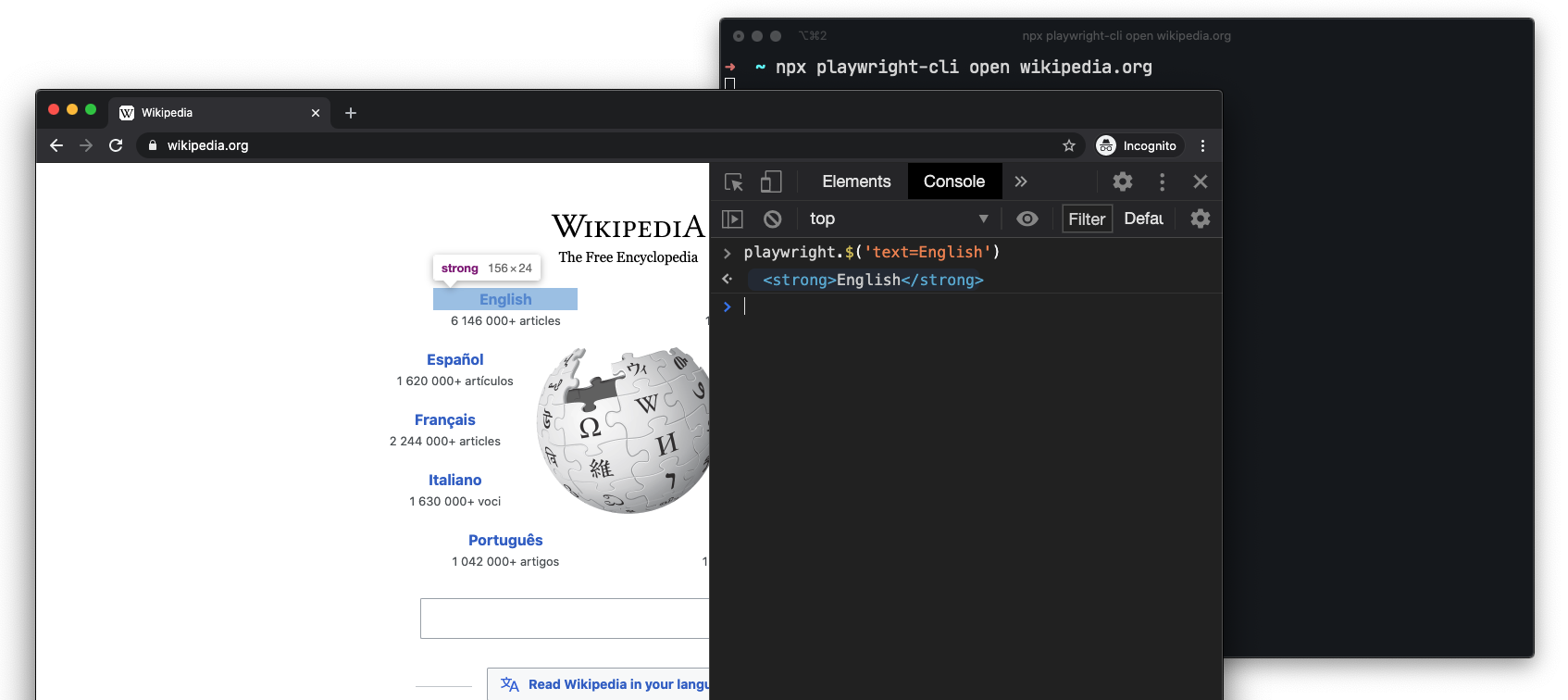
playwright.$(selector)
Query Playwright selector, using the actual Playwright query engine, for example:
> playwright.$('.auth-form >> text=Log in');
<button>Log in</button>
playwright.$$(selector)
Same as playwright.$
, but returns all matching elements.
> playwright.$$('li >> text=John')
> [<li>, <li>, <li>, <li>]
playwright.inspect(selector)
Reveal element in the Elements panel (if DevTools of the respective browser supports it).
> playwright.inspect('text=Log in')
playwright.locator(selector)
Query Playwright element using the actual Playwright query engine, for example:
> playwright.locator('.auth-form', { hasText: 'Log in' });
> Locator ()
> - element: button
> - elements: [button]
playwright.highlight(selector)
Highlight the first occurrence of the locator:
> playwright.highlight('.auth-form');
playwright.clear()
> playwright.clear()
Clear existing highlights.
playwright.selector(element)
Generates selector for the given element.
> playwright.selector($0)
"div[id="glow-ingress-block"] >> text=/.*Hello.*/"
Verbose API logs
Playwright supports verbose logging with the DEBUG
environment variable.
- Bash
- PowerShell
- Batch
DEBUG=pw:api npx playwright test
$env:DEBUG="pw:api"
npx playwright test
set DEBUG=pw:api
npx playwright test